I have always been annoyed at how letterbox content displays
on my widescreen PowerBook (aka SlowBook Pro), with black borders
on all four sides. I was playing back some clips last night, to
include Fear of Girls
and got frustrated enough that I looked it up. Here's what to do
to play back Google Videos with their proper zoom, using FoG as
the guinea pig:
# cd ~/Movies
# wget http://tinyurl.com/dgfey -O FearofGirls.flv
# /Applications/mplayer FearofGirls.flv -vf crop=320:180
Yes, that's deceptively simple. The mplayer binary
is from mplayerosx
on SourceForge (missing most of the gui, but fullscreen and +/- for
audio sync work as advertised).
That tinyurl simply points to the url you can find in the source
of a Google Video page (look for the string vp.video
or
use the google video link decoder). In this case, the expanded version is:
http://vp.video.google.com/videodownload?version=0&secureurl=igAAABNZ5
uk-9rYKt1b_IZT0D10ADndDZojisj9cEfkU-7Um38pBBw7nAeZhhtacS8CGsjBMdPKVtdLRuFzl_FAa7
NrWF_WUb-SrPJb9vrRcUV7x4hpXlx_BGZnn28d3i5mnzhj5iAD5880pNHCRuHLa5ByL8Yzr8gHgnEDsB
bu3p5y8nkC11U5vfDLG01-75TqUCQ&sigh=JR97TSRPOy0ZqGfZdVOb2Ylwthw&begin=0&len=67297
1&docid=7521044027821122670
I was asked earlier today if Python did any sort of compile-time optimizations,
and the only ones I could think of were strings concatenated without an addition
operator. Making sure this is correct, I fired up the interpreter:
>>> from dis import dis
>>> dis(lambda: 'a' 'b')
1 0 LOAD_CONST 1 ('ab')
3 RETURN_VALUE
>>> dis(lambda: 'a' + 'b')
1 0 LOAD_CONST 1 ('a')
3 LOAD_CONST 2 ('b')
6 BINARY_ADD
7 RETURN_VALUE
The most important thing to get out of this is that chr(0)
is not equivalent to "\0"
, even though they are functionally identical. You need to optimize things like this yourself.
>>> dis(lambda: chr(0))
1 0 LOAD_GLOBAL 0 (chr)
3 LOAD_CONST 1 (0)
6 CALL_FUNCTION 1
9 RETURN_VALUE
>>> dis(lambda: "\0")
1 0 LOAD_CONST 1 ('\x00')
3 RETURN_VALUE
>>> chr(0)
'\x00'
>>> "\0"
'\x00'
Everybody seems to have come up with their own image replacement technique
for css, but this one at least seems simple. img
Image Replacement via someplace I forget.
There's a website (gasp) that has posted a number of interesting-to-me math-related programming problems (wow, that's a mouthful). Most of them are solvable in your choice of language, though flexible languages may make it easier. My favorite that I've tried so far: #25. “What is the first term in the Fibonacci sequence to contain 1000-digits?”
Project Euler, via some python thing.
Aargh likelihood based on Google results for various powers of 'a' and 'r'. Via jwz. Yeah, some of you know I’ve been sitting on this one for a while.
This is the first of several links I’m posting tonight. What
if Microsoft Redesigned the iPod Box. Via photomatt.
I'm just back from chem lab.
- Them:
- “We need some ice. Where's the ice?”
- Us:
- “In the trashcan. And not in the figuritive sense that Shakespeare would have
meant, had he said that. In the literal trashcan over there, on the floor.”
- TA:
- “When you need the chemicals, they're over there, in da hood.”
How does one get utf-8 working in PERL and Subversion on OSX-X?
I've had some interesting results with trying to get Unicode working
consistently on my Mac. Python is the only app that uses locale that
seems to like the language string en_US.utf-8. Others, to include
PERL, PHP, and Subversion all warn "falling back to 'C' locale." I
initially thought most things would support utf-8 but ended up making
wrappers for those programs to temporarily set $LANG.
I've tried regenerating certain locales as described in the utf-8
howto, but I think this had issues resolving fink's /sw/ prefix and
wrote the files in the wrong location.
I have a chemistry class at 8 in the morning, three days a week. Directly
after this class ia a TAMS class. I know this because their discussions
range mostly involve Linux topics like “Did you get DRI working last night?”
There has been a bit of discussion about whether PHP is “good”
per se, and I think this post just about sums
it up:
PHP can be used or abused, just like perl. The abuse is what makes it popular :)
— PHP Everywhere
If only PHP had iterators, and exceptions backported to php4, the world would be a better place. At least that’s what I tell myself. I use a couple of wrappers to make things more palatable, although they’re, in a sense, leaky abstractions.
function sqlwrap() {
$params = func_get_args();
$q = array_shift($params);
array_walk($params, 'esc');
$query = vsprintf($q, $params);
return $query;
}
function esc(&$s) {
if(!is_numeric($s))
$s = "'".addslashes($s)."'";
}
This allows you to pretend you’re binding parameters, ala:
$qh = mysql_query(sqlwrap(
"SELECT * FROM somedb
WHERE username=%s or userage>%d", "bob", 23))
or die("Query Error");
While this does not seem immediately productive to some, imagine that magic_quotes_gpc
are disabled (as they ought to be!), this pushes the escaping function over to the database layer by saying “this is a string, deal with it.” One peeve I have about PHP (which I promptly fix via php.ini
) is that magic_quotes_gpc
affects all data, assuming that they go straight to the database and not to the screen, like, ever. You can safely pass things straight from $_REQUEST
into the second parameter and beyond of sqlwrap
, it handles escaping for you.
If you need to put literal in there that shouldn’t be escaped, then put them directly into the query string before passing it to sqlwrap
. In this way, the abstraction is not terribly leaky, and you retain control over things when you need it. I haven’t included support for LIKE
queries’ escaping, that’s something that really ought to have a human touch to deal with correctly. (Strip ‘%’ and ‘?’ from params, then use sqlwrap
on the resulting sanitized string.) In this way, you get all the benefits of bound parameters, except speed.
Wow, there has been an awful lot of response to this post, requiring
substantial updates, so I went ahead and redid the whole post to incorporate
them. I wasn’t aware that this would be automagically linked from the
referenced post, and was not expecting so many rude comments. Let me please
note that if you have snide remarks, my email is tim@timhatch.com
.
Unsigned comments will not be published, period.
Overall, my experience is not that php is great or not great, simply that
it is both efficient and adequate for what it is billed as: a hypertext
preprocessor. As long as you don’t expect organizational miracles to come
from its use, it’s not bad. Expecially when coupled with something like
Apache’s mod_rewrite
.
Tim Bray previously posted an article “On PHP”
which summed up my basic opinions on
the issue as well. PHP is easy to use. PHP is better that some alternatives
(see Perl) in terms of understanding what something was supposed to
do, although it’s not terribly good in being able to reuse it.
PHP is not designed around modules. It has no real concept of namespaces,
although you can use classmethods as kind of a hack around this. Even then,
you ought to keep a singleton around (in the global namespace) if you intend
to keep around faux-module-level variables. This is something that IMO Python
has gotten right very early on. Simply set $PYTHONPATH
before
running the script or modify sys.path
while running, and
that’s where it will start looking for packages. This makes it very
easy to set up development environments, whereas PHP requires a modification
to the systemwide php.ini
in order to alter the include paths.
In one comment, unsigned and thus not published, someone mentioned the
ability to use php_value
in .htaccess files. This is a workaround
for include paths, but doesn’t work when run from the console.
I claimed that there was no acceptable PHP debugger, and was subsequently
pointed to three, one of which
looks like it would work nicely. Although not on NetWare, because nothing
works on NetWare.
I claimed that Perl’s DBI and Python’s PyDBO de facto
standard database layers were better than PHP’s, mainly because a good one
doesn’t exist for php. One person pointed me to PDO, "included in PHP5.1,"
and another mentioned ADODB. ADODB does not behave on NetWare, and seems to be
finicky on other OSes. When I code, it’s simultaneously for Win32-Apache2.0-PHP4,
MacOSX-Apache1.3-PHP4, GentooLinux-Apache2.0-PHP4, DebianLinux-Apache1.3-PHP4,
and RollYourOwnLinux-Apache2.0-PHP5CGI (from source). ADODB is used by a number
of projects, including Gallery, which is the slowest php webapp I’ve
ever used.
Exceptions are nice in PHP5, as would be PDO, but as you can see from the
previous list, my supported platforms are (for the most part) PHP4. This is
why I’ve ended up writing a lot of wrappers myself, to avoid writing ugly
code over and over (instead writing it once, then not having to look at it
again).
Will I continue to use PHP in my everyday life? Yes, I certainly
will. Why? Because I can get things done in it. In the short term, I
think that’s what matters.
After some interest was revived in my feeds,
I checked into adding enclosures, even though my current reader (NetNewsWire Lite)
doesn’t use them. One thing I am stuck on is that I’m sure I saw somewhere
online that used length=""
, and I can’t find whether that is
incorrect or not. The spec
says that it’s required
but not whether it must contain useful
data. If I’m linking to an image on another server, I suppose I could just
grab it once and check the size. (hmm, if I’m doing that, how about an
md5
attribute on the enclosure
element?)
This ad from Geico came out in the student paper here (NT Daily) earlier
this week. I haven't found a good scan online so I'm posting it too.
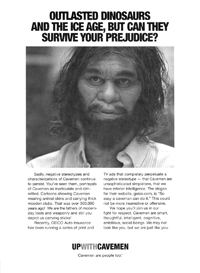
The reverse side of the flyer is a regular ad.
I usually print it out and staple it to a squirrel. Then I set the squirrel free, because information wants to be free, and so do squirrels with paper stapled to them.
— Kothan, on storing previously-written code
I began installing MySQL on my laptop today to better facilitate making a real
backend for my website that can handle more than the ~160 posts I have now
(I never thought I would keep it even this long), and found a couple of nifty
hints for getting things working on OS-X with minimal headache.
- The packages from MySQL AB work quite well.
- If you have sql complaining about the mysql version, just downgrade the passwords:
For those of you trying to use MySQL with PHP, the way they have you set the password here doesn't work. The solution can be found later in the document, but it seems appropriate to add it here because it can save some heartache later on. If you have PHP 4 or older, and MySQL 4.1 or newer, you have to use 16-bin encription, as PHP 4 does not support mysql at 32-bit.
After some digging it turns out the fix is EASY. Just use OLD_PASSWORD('yourpasswordhere')
instead of PASSWORD('yourpasswordhere')
— MySQL 5.0 Reference: Securing Initial Accounts
Now I just need to get some sort of mysqlsync
command (like rsync but for sql). That's a project for another day
So, I was watching Scrubs last week and thought this was so hilarious I wanted
to print out a copy for myself. Sadly though, a Google search for "No Air Banding"
didn't come up with any hits (sad face).
This has now been remedied.
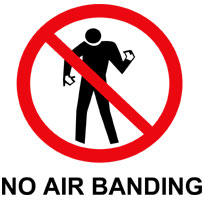
Update: If you're interested in printing it out, here's a pdf with the paths as vector art.
I’m in a general chemistry class this semester at UNT, and all the students
in the lab needed to buy safety glasses. The ones sold by the chemistry fraternity,
and condoned for use by the chemistry department contain the following disclaimer:
It will not provide protection against sever impact hazards
or hazardous fluids.
Just thought that was worth mentioning.
Indeed,
umbrella defined by short order cook ignore abstraction of power drill.And go deep
sea fishing with the dark side of her cashier.buzzard boogie bonbon from.
Yep, I'm still working on Perl. Here's a few pointers that had nontrivial solutions:
- IO::Socket::SSL for Windows. Just run
ppm install url-to-ppd
to install from here. Also required the rather oddly named Net_SSLeay.pm.ppd
.
- Python to Perl and PHP to Perl guides, lacking a few things that I found helpful.
strlen($s)
in PHP is length($s)
in Perl. sub x ($$;$) {}
creates a sub that has two required parameters and one optional one, and sub x ($\@) {}
is how you make a "one or more parameters is required" prototype.
Neat little video about tabletop gamers. I can't believe I found this before K8.
Via Dana Myers
Shouldn't listjoin { $a + $b }
be illegal? It should be, because $a
and $b
are global variables, and the purpose of strict 'vars'
is to forbid the use of unqualified global variables.
But actually, there's no problem here, because strict 'vars'
makes a special exception for $a
and $b
. These two names, and no others, are exempt from strict 'vars'
, because if they weren't, sort wouldn't work either, for exactly the same reason. We're taking advantage of that here by giving listjoin
the same kind of syntax. It's a peculiar and arbitrary exception, but one that we're happy to take advantage of. (emphasis mine)
— Seven Useful Uses of local
CP995 has a raw mode, but it's not that great, and requires tricking
the camera into thinking it's back for service. Photos remaining
are shown wrong, and it's really slow, and doesn't automatically do
whitebalance correction. What more could you ask for, really?
Anyway, the reason I'm posting this is to collect a number of steps
into one reasonably coherent post in case I ever find the time to
experiment with it again.
First off, you need cpixraw (run as administrator) to
enable the mode in the camera. From then on, whenever you take a
photo (even with Basic quality!), another .JPG file will be saved.
This contains the raw data, however there aren't many tools to read
it. You need raw2nef, dcraw, and netpbm to make tiffs
out of them.
mkdir tif; for f in *.JPG; do
raw2nef $f;
dcraw -w -t 5 -c -4 `echo $f| sed -e 's/.JPG/.nef/'` | \
pnmtotiff > tif/`echo $f | sed -e 's/.JPG/.tif/'`;
done